if i have datafram to display on how will my template looks like?
This is an example of how you can render a Pandas DataFrame in a Flask template:
<!doctype html>
<html>
<head>
<title>Stocks Information</title>
</head>
<body>
<h1>Stocks Information</h1>
<table>
<thead>
<tr>
<th>Symbol</th>
<th>Indicator 1</th>
<th>Indicator 2</th>
<th>Indicator 3</th>
</tr>
</thead>
<tbody>
{% for index, row in df.iterrows() %}
<tr>
<td>{{ row['Symbol'] }}</td>
<td>{{ row['Indicator 1'] }}</td>
<td>{{ row['Indicator 2'] }}</td>
<td>{{ row['Indicator 3'] }}</td>
</tr>
{% endfor %}
</tbody>
</table>
</body>
</html>
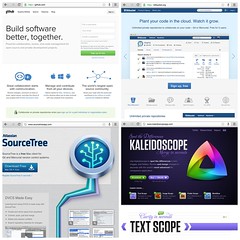

This template includes a table that displays the data from a Pandas DataFrame. The DataFrame is passed to the template as a variable named df
. The template uses a for
loop to iterate over the rows of the DataFrame and generate a row in the table for each row of the DataFrame.
To render the template in your Flask application, you can use the render_template()
function and pass the DataFrame as a keyword argument. For example:
from flask import Flask, render_template
import pandas as pd
app = Flask(__name__)
@app.route('/')
def home():
df = pd.read_csv('data.csv')
return render_template('stocks.html', df=df)
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
This Flask application reads a CSV file containing the stocks information and loads it into a Pandas DataFrame named df
. The df
variable is then passed to the stocks.html
template using the render_template()
function.
I hope this helps!